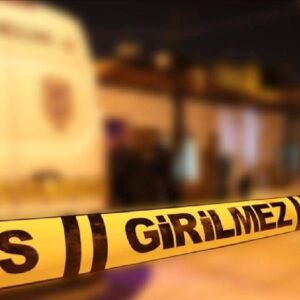
Arazi kavgasında silahlar konuştu: İki yaralı
Diyarbakır’da arazi anlaşmazlığı nedeniyle çıkan kavgada iki kişi yaralandı.
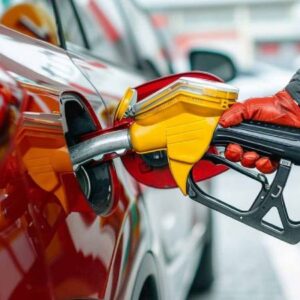
Akaryakıtta son durum: İşte güncel benzin, motorin ve LPG fiyatları
Akaryakıtta son durum: İşte güncel benzin, motorin ve LPG fiyatları
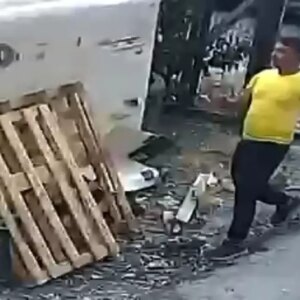
Avcılar’da Palet Hırsızlığı
Mobilya mağazasından beş palet çalan şüpheli güvenlik kameralarına yakalandı.
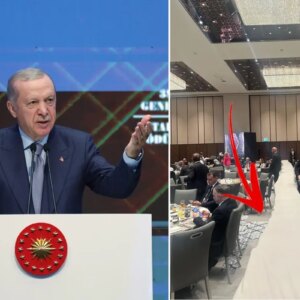
Cumhurbaşkanı Erdoğan’ın da katıldığı DEİK galasında iş adamlarının tepkisini çeken oturma düzeni
Dış Ekonomik İlişkiler Kurulu’nun 40. yıl gala gecesindeki oturma düzeni, katılan iş adamlarını rahatsız etti. Branda ile ikiye bölünen salonda bir bölüm “protokol kartı” taşıyan davetlilere ayrılırken, diğer bölümdeki konukların bu alana geçişine izin verilmedi.
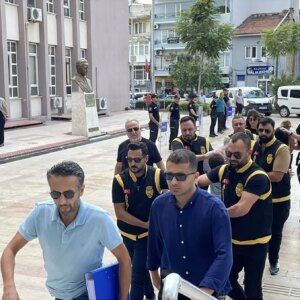
Üniversite Öğrencisi Cinayeti: 1 Tutuklama
Aydın’da üniversite öğrencisi Teslime Hanedan’ın cinayetiyle ilgili 1 kişi tutuklandı, 4 serbest.